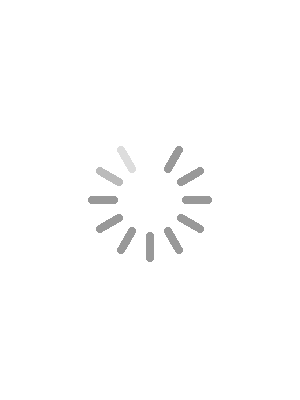
Hintergrund-Bild hochladen
Sie können hier ein eigenes Foto als Hintergrund für Ihren Showoom hochladen.
Hintergrund-Bild löschen
Entfernen Sie hier ein selbst hochgeladenes Bild wieder.
Hintergrund-Bild verkleinern oder vergrößern
Hier können Sie die Darstellungsgröße Ihres Hintergrund-Bildes im Showroom verändern.
Artikel verkleinern oder vergrößern
Hier können Sie die Darstellungsgröße aller Artikel im Showroom verändern.
Showroom drucken
Button zum Ausdrucken Ihres aktuellen Showrooms.
Showroom herunterladen
Hier können Sie Ihren aktuellen Showroom als Bild im JPG-Format herunterladen.
Artikel verschieben
Hier können Sie einen Artikel im Showroom positionieren.
Artikel spiegeln
Hier können Sie einen Artikel horizontal spiegeln (z.b. bei Leuchtern mit einer Schräge).
Artikel duplizieren
Hier können Sie einen Artikel ein zweites mal im Showroom platzieren.
Einzelnen Artikel in den Warenkorb legen
Sie werden zur Artikelansicht geführt, wo Sie die Artikeloptionen auswählen den Artikel "in den Warenkorb" legen können.
Artikel löschen
Hiermit löschen Sie einen Artikel aus dem Showroom.